Q14. Discuss about the accessing of Base class members.
Answer:
The base class members can be accessed by its sub-classes through access specifiers. There are three types of access specifies. They are public, private and protected.
1. Public
When the base class is publicly inherited, the public members of the base class become the derived class public members.
They can be accessed by the objects of the derived class.
2. Protected
When the base class is derived in protected mode, the ‘protected’ and ‘public’ members of the base class become the protected members of the derived class.
Private and protected members of a class can be accessed by,
(i) A friend function of the class.
(ii) A member function of the friend class.
(iii) A derived class member function.
Syntax
The base class members can be accessed by its sub-classes through access specifiers. There are three types of access specifies. They are public, private and protected.
1. Public
When the base class is publicly inherited, the public members of the base class become the derived class public members.
They can be accessed by the objects of the derived class.
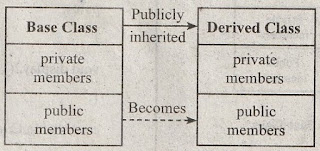
Figure: Publicly Inherited Base Class
Syntax
class classname
{
public:
datatype variablename;
returntype functionname();
};
When the base class is derived in protected mode, the ‘protected’ and ‘public’ members of the base class become the protected members of the derived class.
Private and protected members of a class can be accessed by,
(i) A friend function of the class.
(ii) A member function of the friend class.
(iii) A derived class member function.
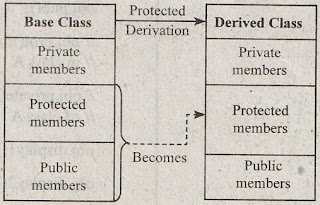
Figure: Protected Derivation of the Base Class
Syntax
class classname
{
protected: :
datatype variablename;
returntype functionname();
};
3. Private
When a base class contains members, that are declared as private, they cannot be accessed by the derived class objects. They can be accessed only by the class in which they are defined.
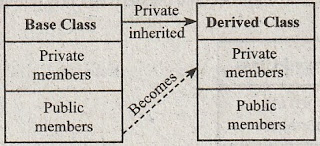
Figure: Privately inherited Base Class
Syntax
class classname
{
private:
datatype variablename;
returntype functionname( );
};
#include <iostream>
using namespace std;
class A
{
public: pub()
{
cout << “In Public() \n”;
}
protected: prot()
{
cout << “In Protected() \n”;
}
private: priv()
{
cout << “In Pivate() \n”;
}
};
class C : public A
{
public:
void display1()
{
cout<< “In C::display1
call\n”;
pub();
}
void display2()
{
cout << “In C::display2
call\n”;
prot();
}
/*pri(Q is a private member of
class A. Therefore it is an illegal access
void display3()
{
cout<<“In C::display3
call\n”;
priv();
} */
}
main()
{
C obj;
obj.pub();
// obj.prot(); illegal because
it is declared as protected in class A
// obj.private(); illegal
because pri() isa private member of class A
obj.display1();
obj.display2();
}
Output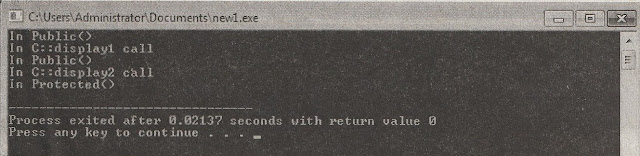