Q17. What are static members of a class? Explain.
Answer:
Static Members of a Class
The static members of a class are static member function and static member variable.
(i) Static Member Function
When a member function is preceded by static keyword then it is called as static member function. The static member the functions have the ability to access static member variables and the member functions of the same class. These static member functions are invoked by the class name without using the class object. The scope of static member is valid in the entire class, but, it does not create any side effects to other part of the program.
There are few important points to remember while using static member functions. They are as follows:
- For static members, memory is allocated only once to entire class and all the class members will share the copy of the memory.
- The static member function is invoked by class name, followed by function name and terminated with semicolon. The class name and function name are separated by scope resolution operator (::).
- The static member functions are also invoked by using objects.
Example
#include<iostream.h>
#include<conio.h>
class Number
{
private:
static int result; //static member
variable declaration
static int a, b; //declaration of
static member variables
public:
static void add( ) //definition SE
static member function
{
result=a+b;
}
static void display( )
{
cout<<“The numbers
are:”<<a<<“\t”<<b<<endl;
cout<<“The sum
is:”<<result;
}
};
int number::result=0;
int number::a=5;
int number::b=6;
int main()
{
clrscr( );
number::add( ); //calls add
function
number::display( ); Heals display
function
getch();
return 0;
}
Output

(ii) Static Member Variable
A member variable that is preceded with the static keyword is called as static member variable. In a class, memory is allocated to all the objects and member variables are assigned to all objects. There is a possibility for member variables to allocate memory like member functions. This can be done by using the keyword static.
The static variables that are declared in the class have limited access within the class, but they are alive till the execution of the program is completed. When a local variable is declared as static it maintains last variable’s value.
Syntax
static<definition of
variable>
There are some reasons to declare static member variables outside the class. They are as follows:
1. The memory is allocated separately to static member variables irrespective of objects.
2. The aah member variables must be initialized with a value to avoid linker errors.
3. For the static member variables, memory is allocated at most one time.
4. The memory is allocated only once for static variables and the entire class objects will use the common static variable.
Program
#include<iostream.h> .
#include<conio.h>
class example
{
static int x; //declaration of
static variable
int y; //declaration of non-static
variable
public:void null( )
{
y=8;
}
void total( )
{
x--;
y--;
cout<<“\n The. x value
is:”<<x<<“\t’<<“\n Address of
x="<<(unsigned)&x;
cout<<“\n The y value
is:’<<y<<“\t”<<“\n Address of
y="<<(unsigned)&y;
}
};
int example::x=5; //initialization
of static member variable
int main()
{
example exl, ex2;
ex1 null();
ex2.null();
exl.total();
ex2.total()
getch();
return 0;
}
Output
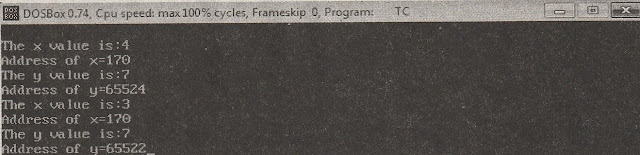