Q30. Explain about structures in detail.
Answer:
Structure
A structure is an aggregate datatype which can hold objects of various sizes and types. It is a user defined data type. Objects of a structure can be data or functions. The access level for structure members is public by default where as the access level for class members is private.
Structure Declaration
A structure can be defined as follows,
struct Employee
{
int id;
char name[10];
char dept[20];
float salary;
};
The above structure has four
members id, name, dept and salary.
The keyword ‘struct’ is used to define a structure. The memory is not allocated upon structure declaration. It is only a blue print for variable creation.
Defining a Structure Variable
A structure variable can be defined in main( ) once the structure is declared. The general format for defining a structure is as follows, :
Employee e;
In the above statement, e is a variable of type structure Employee. The compiler allocates the memory for the structure members, when structure variable is defined.
Accessing Structure Members
The structure members can be accessed using the dot operator. The general format for accessing structure members is as follows,
e.name;
e.salary = 25000;
Example
#include<iostream.h>
#include<conio.h>
struct Employee
{
int id;
char name[20];
char dept[20];
float salary;
};
int main()
{
Employee e;
cout<<“Enter Employee
id:\n”;
cin>>e.id;
cout<<“Enter Employee
Name:\n”;
cin>>e.name;
cout<<“Enter Department:\n”;
cin>>e.dept;
cout<<“Enter salary:\n”;
cin>>e.salary;
cout<<“Employee
Information:”;
cout<<“Employee
id:”<<e.id<<endl;
cout<<“Employee
Name:”<<e.name<<endl;
cout<<“Department:”<<e.dept<<endl;
cout<<"salary:"<<e.salary<<endl;
getch( );
return 0;
}
Output
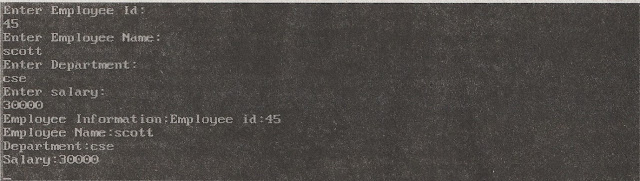