Q29. List and explain string manipulation functions.
Answer:
String Manipulation Functions
C++ provides a set of string handling functions to work on strings. These functions are available in string.h header files. The following are few string manipulation functions. They are,
- strlen() function
- strcpy() function
- strcat() function
- strcmp() function
- strchr() function
- strstr() function
- substr() function
strlen() function returns the length of the string. It does not include the null terminator.
Syntax
strlen(string1);
Example
strlen(“Hello”);
2. strcpy() Function
strcpy() function is used to copy a string to a string variable.
Syntax
strcpy(string1,
string2);
Example
strcpy(name,
“helloworld”);
Here, both string1 and string2 are character arrays where, string2 may be a string variable (or) string constant.
3 strcat() Function
strcat() function appends one string at the end of another string. It returns a single string that consists of characters of string1 followed by string2.
Syntax
strcat(string1,
string2);
Example
strcat(“Hello”,
“World”);
4. strcmp() Function
strcmp( ) function is used to compare two strings. It returns 0 if string1 and string2 are equal, If string1 is greater than string2 it returns negative number and if string2 is less than string 1, it returns positive number.
Syntax
strcmp(string1, string2);
Example:
strcmp(‘“Hello”, “Help”);
5. strchr() Function
strchr( ) function finds the first occurrence of given character in a string.
Syntax
char strchr(const
char str, int ch);
Example
strchr(welcome,
e);
6. strstr() Function
strstr() function returns a pointer to first occurrence of string2 in string1.
Syntax
strstr(string1,
string2);
Example
strstr(“estudies”,
“foryou”);
7. substr() Function
substr( ) extracts the characters between two specified indices from a string and returns the new sub string.
Syntax
str.substr(start,
end);
Example
str = “Hello
world”
str.substr(2, 5);
Program
#include<iostream>
#include<cstring>
using
namespace std;
int
main( )
{
int x,
lenl, len2;
char
str1[] = “siagroup”;
char
str2[] = “group”;
cout<<“\nstrlen(
)”;
len1=
strlen(str1);
len2=
strlen(str2);
cout
<<“\nstr1:”<<strl<< “\nlength
<<lenl<<“characters”<< endl;
cout
<<“\nstr2: ”<<str2<<“\nlength:
»<<len2<<“characters”<<endl;
cout<<“\nstrcmp()”;
x=
strcmp(str1, str2);
if(x!=0)
{
cout<<“\n\nStrings
are not equal \n”;
cout<<“\nstrstr()”;
char
*p = strstr(str1,str2);
if
(p)
cout<<
“\n” <<str2 <<“ is present in ” <<strl <<“ at position”
<<p-str1;
else
cout<<“\n”
<<str2<<“ is not present ” <<str1<<endl;
cout<<“\nstrcat()”;
strcat(str1,
str2);
cout<<“\nstr1
=\t ’<<str1; /*joining strl and str2*/
}
else
cout<<“\n\nStrings
are equal \n”;
char*
ch = strchr(str1,'i’);
cout<<“\n
strchr()”;
cout<<“\n’<<ch
- str1+1;
strcpy(str1,
str2);
cout<<“\nstrcpy(
)”;
cout<<“\nstr1 = \t’<< str1;
return
0;
}
Output
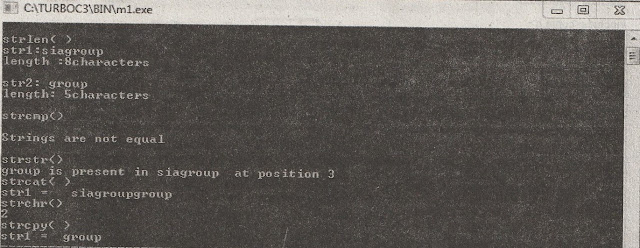