4. Hierarchical Inheritance
It refers to the process of deriving many classes from a single base class. All the derived classes can be further inherited by some other classes in the same way.
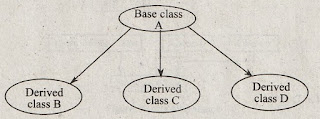
Figure: Hierarchical Inheritance
Syntax
class A
{
--------
};
class B: visibility A
{
--------
};
class C: visibility A
{
--------
}
class D: visibility B
{
--------
};
Example
class Automobiles
{
// body
};
class Cars : public Automobiles
{
// body
};
class Bikes :; public Automobiles
{
// body
};
Program
#include <iostream>
using namespace std;
class A
{
public:
int x;
void get()
{
cout << “Enter value of x:”;
cin >> x;
}
};
class B : public A
{
public:
void Square()
{
cout << “The square of the
given number is: ” <<x * x;
}
};
class C : public A
{
public: :
void cube()
{
cout << “\nThe cube of the
given number is: ” << x * x *x;
}
};
int main()
{
B obl;
C ob2;
ob1.get();
ob1.Square();
ob2.cube();
return 0;
}
Output
