3. Multi-level Inheritance
It refers to the process of deriving a class from another derived class as shown in the figure below,
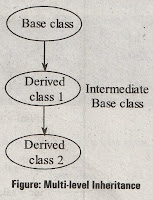
Figure: Multi-level Inheritance
Syntax
class BaseClass
{
-----
};
class DerivedClass1 : Visibility
BaseClass
{
-----
};
class DerivedClass2 : Visibility
DerivedClass1
{
-----
};
Example
class Automobiles
{
// body
};
class Cars : public Automobiles
{
//body
};
class Mercedez : public Cars
{
//body
};
#include<iostream.h>
#include<conio.h>
class Employee
{
protected:
int id;
public:
void set_id(int);
void show_id(void);
};
void Employee::set_id(int x)
{
id =x;
}
void Employee::show_id(void)
{
cout <<“Employee-id
is:"<<id<<endl;
}
class Salary:public Employee
{
protected:
float sal;
public:
void setSal(float);
void showSal(void);
};
void Salary::setSal(float S)
{
sal = S;
}
void Salary::showSal(void)
{
cout<<“Employee salary
is:”<<sal<<endl;
}
class Increment:public Salary
{
private:
float inc;
public:
void show(void);
};
void Increment: :show(void)
{
if (sal > 10000.00)
{
sal = sal + 1000.00;
inc = sal;
cout<<“Salary after
increment is:”<<inc<<endl;
}
else
{
cout<<“No
increment”<<endl;
}
}
void main()
{
increment i, j;
clrscr();
i.set_id(100);
i.show_id();
i.setSal(15000,00);
i.showSal( );
i.show( );
j.set_id(101);
j.show_id();
j.setSal(9000.00);
j.showSal();
j.show( );
getch();
return;
}
Output
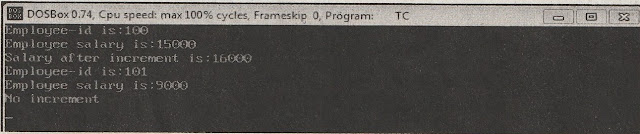